Java Inventory Management System Project
FREE Online Courses: Knowledge Awaits – Click for Free Access!
In this project, we will be creating an Inventory Management system implemented using Java Swing. It provides a graphical user interface (GUI) for managing products, categories, purchasing, and tracking inventory and sales. The application allows users to add new products and categories, assign categories to products, purchase products, and track inventory and sales data. It utilizes a database connection to store and retrieve data related to products, categories, and transactions. The database used is SQLite.
About Java Inventory Management System
The objective of this project is to demonstrate the implementation of an Inventory Management system using Java Swing. It aims to showcase how to create a GUI application that allows users to perform various inventory management operations, such as adding products, and categories, purchasing products, and tracking inventory and sales.
Prerequisites For Inventory Management System Using Java
- Basic understanding of Java programming language
- Familiarity with object-oriented programming concepts in Java
- Knowledge of Java Swing framework for creating GUI applications
- Understanding of databases and SQL for database operations
- Familiarity with handling events and event-driven programming in Java
- Basic knowledge of data structures and algorithms for working with tables and collections of data
- Any Java IDE (Eclipse is used for this Tutorial)
Download Java Inventory Management System Project
Please download the source code of Java Inventory Management System App Project: Java Inventory Management System Project Code.
Steps to Inventory Management System Project Using Java
Step 1: Create the database connection class “DatabaseConnection.java”
Step 2: Creating the AddProductWindow class
Step 3: Create the UI for our application in class “InventoryManagement.java”
Step 1:Creating the database connection class “DatabaseConnection.java”
Here is the complete code for this class:
package org.ProjectGurukul; import java.sql.*; import java.util.List; import javax.swing.JComboBox; import javax.swing.table.DefaultTableModel; public class DatabaseConnection { private Connection connection; // Constructor - Establishes the database connection public DatabaseConnection(String databaseName) { try { // Load the SQLite JDBC driver Class.forName("org.sqlite.JDBC"); // Connect to the specified database connection = DriverManager.getConnection("jdbc:sqlite:" + databaseName); System.out.println("Connected to the database."); } catch (ClassNotFoundException e) { System.out.println("SQLite JDBC driver not found."); } catch (SQLException e) { System.out.println("Failed to connect to the database: " + e.getMessage()); } } // Close the database connection public void closeConnection() { try { if (connection != null && !connection.isClosed()) { connection.close(); System.out.println("Disconnected from the database."); } } catch (SQLException e) { System.out.println("Failed to close the database connection: " + e.getMessage()); } } // Create the database schema for the application public void createSchema() { try { // Create the products table String createProductsTableQuery = "CREATE TABLE IF NOT EXISTS products (" + "id INTEGER PRIMARY KEY AUTOINCREMENT," + "name TEXT NOT NULL," + "quantity INTEGER NOT NULL," + "price REAL NOT NULL," + "created_at DATETIME DEFAULT CURRENT_TIMESTAMP," + "updated_at DATETIME DEFAULT CURRENT_TIMESTAMP)"; Statement createProductsTableStatement = connection.createStatement(); createProductsTableStatement.execute(createProductsTableQuery); createProductsTableStatement.close(); // Create the categories table String createCategoriesTableQuery = "CREATE TABLE IF NOT EXISTS categories (" + "id INTEGER PRIMARY KEY AUTOINCREMENT," + "name TEXT NOT NULL)"; Statement createCategoriesTableStatement = connection.createStatement(); createCategoriesTableStatement.execute(createCategoriesTableQuery); createCategoriesTableStatement.close(); // Create the product_categories table String createProductCategoriesTableQuery = "CREATE TABLE IF NOT EXISTS product_categories (" + "product_id INTEGER," + "category_id INTEGER," + "FOREIGN KEY (product_id) REFERENCES products(id)," + "FOREIGN KEY (category_id) REFERENCES categories(id)," + "PRIMARY KEY (product_id, category_id))"; Statement createProductCategoriesTableStatement = connection.createStatement(); createProductCategoriesTableStatement.execute(createProductCategoriesTableQuery); createProductCategoriesTableStatement.close(); System.out.println("Schema created successfully."); } catch (SQLException e) { System.out.println("Failed to create schema: " + e.getMessage()); } } // Insert a product into the products table public void insertProduct(String name, int quantity, double price) { String query = "INSERT INTO products (name, quantity, price) VALUES (?, ?, ?)"; try { PreparedStatement statement = connection.prepareStatement(query); statement.setString(1, name); statement.setInt(2, quantity); statement.setDouble(3, price); int rowsInserted = statement.executeUpdate(); if (rowsInserted > 0) { System.out.println("Product inserted successfully."); } else { System.out.println("Failed to insert product."); } } catch (SQLException e) { System.out.println("Failed to insert product: " + e.getMessage()); } } // Insert a category into the categories table public void insertCategory(String name) { String query = "INSERT INTO categories (name) VALUES (?)"; try { PreparedStatement statement = connection.prepareStatement(query); statement.setString(1, name); int rowsInserted = statement.executeUpdate(); if (rowsInserted > 0) { System.out.println("Category inserted successfully."); } else { System.out.println("Failed to insert category."); } } catch (SQLException e) { System.out.println("Failed to insert category: " + e.getMessage()); } } // Assign a category to a product in the product_categories table public void assignCategoryToProduct(int productId, int categoryId) { String query = "INSERT INTO product_categories (product_id, category_id) VALUES (?, ?)"; try { PreparedStatement statement = connection.prepareStatement(query); statement.setInt(1, productId); statement.setInt(2, categoryId); int rowsInserted = statement.executeUpdate(); if (rowsInserted > 0) { System.out.println("Category assigned to product successfully."); } else { System.out.println("Failed to assign category to product."); } } catch (SQLException e) { System.out.println("Failed to assign category to product: " + e.getMessage()); } } // Update the quantity of a product for a purchase public void purchaseProduct(int productId, int quantityToPurchase) { String query = "UPDATE products SET quantity = quantity + ? WHERE id = ?"; try { PreparedStatement statement = connection.prepareStatement(query); statement.setInt(1, quantityToPurchase); statement.setInt(2, productId); int rowsUpdated = statement.executeUpdate(); if (rowsUpdated > 0) { System.out.println("Product purchased successfully."); } else { System.out.println("Failed to purchase product."); } } catch (SQLException e) { System.out.println("Failed to purchase product: " + e.getMessage()); } } // Retrieve all products public void getAllProducts(DefaultTableModel tableModel) { String query = "SELECT * FROM products"; tableModel.setRowCount(0); try { Statement statement = connection.createStatement(); ResultSet resultSet = statement.executeQuery(query); while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); int quantity = resultSet.getInt("quantity"); double price = resultSet.getDouble("price"); String createdAt = resultSet.getString("created_at"); String updatedAt = resultSet.getString("updated_at"); tableModel.addRow(new Object[]{id, name, quantity, price, createdAt, updatedAt}); } resultSet.close(); statement.close(); } catch (SQLException e) { System.out.println("Failed to retrieve products: " + e.getMessage()); } } // Retrieve products by category public void getProductsByCategory(int categoryId,DefaultTableModel tableModel) { String query = "SELECT p.* FROM products p " + "JOIN product_categories pc ON p.id = pc.product_id " + "WHERE pc.category_id = ?"; tableModel.setRowCount(0); try { PreparedStatement statement = connection.prepareStatement(query); statement.setInt(1, categoryId); ResultSet resultSet = statement.executeQuery(); while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); int quantity = resultSet.getInt("quantity"); double price = resultSet.getDouble("price"); String createdAt = resultSet.getString("created_at"); String updatedAt = resultSet.getString("updated_at"); tableModel.addRow(new Object[] {id,name,quantity,price,createdAt,updatedAt}); } resultSet.close(); statement.close(); } catch (SQLException e) { System.out.println("Failed to retrieve products by category: " + e.getMessage()); } } // Delete a product by ID public void deleteProduct(int productId) { String query = "DELETE FROM products WHERE id = ?"; try { PreparedStatement statement = connection.prepareStatement(query); statement.setInt(1, productId); int rowsDeleted = statement.executeUpdate(); if (rowsDeleted > 0) { System.out.println("Product deleted successfully."); } else { System.out.println("Failed to delete product. Product not found."); } } catch (SQLException e) { System.out.println("Failed to delete product: " + e.getMessage()); } } // Delete a product by ID public void deleteCategory(int categoryId) { String query = "DELETE FROM categories WHERE id = ?"; try { PreparedStatement statement = connection.prepareStatement(query); statement.setInt(1, categoryId); int rowsDeleted = statement.executeUpdate(); if (rowsDeleted > 0) { System.out.println("Product deleted successfully."); } else { System.out.println("Failed to delete product. Product not found."); } } catch (SQLException e) { System.out.println("Failed to delete product: " + e.getMessage()); } } // Retrieve all categories public void getAllCategories(DefaultTableModel tableModel) { String query = "SELECT * FROM categories"; tableModel.setRowCount(0); try { Statement statement = connection.createStatement(); ResultSet resultSet = statement.executeQuery(query); while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); tableModel.addRow(new Object[]{id,name}); } resultSet.close(); statement.close(); } catch (SQLException e) { System.out.println("Failed to retrieve categories: " + e.getMessage()); } } // Retrieve all ID and names public void updateComboBox(JComboBox<String> cbx) { String query = "SELECT id,name FROM categories"; try { Statement statement = connection.createStatement(); ResultSet resultSet = statement.executeQuery(query); while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); cbx.addItem(id+"|"+name); } resultSet.close(); statement.close(); } catch (SQLException e) { System.out.println("Failed to retrieve categories: " + e.getMessage()); } } // Metthod to sell multiple products public String sellMultipleProducts(List<String> productIds, List<String> quantities) { if (productIds.size() != quantities.size()) { return "Number of product IDs must match the number of quantities."; } String updateQuery = "UPDATE products SET quantity = quantity - ? WHERE id = ?"; try (PreparedStatement updateStatement = connection.prepareStatement(updateQuery)) { try { // Iterate over the product IDs and quantities for (int i = 0; i < productIds.size(); i++) { int productId = Integer.valueOf(productIds.get(i)); int quantityToSell = Integer.valueOf(quantities.get(i)); // Update the quantity of the product updateStatement.setInt(1, quantityToSell); updateStatement.setInt(2, productId); updateStatement.executeUpdate(); } } catch (NumberFormatException e) { e.printStackTrace(); return "Only integer values are allowed"; } return "Products sold successfully."; } catch (SQLException e) { return "Failed to sell products: " + e.getMessage(); } } }
Here’s a brief explanation of each function in the provided code:
1. “DatabaseConnection”: This is the constructor of the “DatabaseConnection” class. It establishes a connection to an SQLite database by loading the JDBC driver and specifying the database name.
2. “closeConnection”: This method is used to close the database connection. It checks if the connection is not null and not already closed, then closes it.
3. “createSchema”: This method creates the database schema for the application. It executes SQL queries to create three tables: “products”, “categories”, and “product_categories”.
4. “insertProduct”: This method inserts a new product into the “products” table. It takes the product name, quantity, and price as parameters and executes an SQL “INSERT” query to add the product to the table.
5. “insertCategory”: This method inserts a new category into the “categories” table. It takes the category name as a parameter and executes an SQL “INSERT” query to add the category to the table.
6. “assignCategoryToProduct”: This method assigns a category to a product in the “product_categories” table. It takes the product ID and category ID as parameters and executes an SQL “INSERT” query to create a new entry in the “product_categories” table.
7. “purchaseProduct”: This method updates the quantity of a product for purchase. It takes the product ID and the quantity to be purchased as parameters and executes an SQL “UPDATE” query to increase the product’s quantity in the “products” table.
8. “getAllProducts”: This method retrieves all products from the “products” table and populates them into a “DefaultTableModel” object for displaying in a table.
9. “getProductsByCategory”: This method retrieves products belonging to a specific category from the “products” table based on the provided category ID. The retrieved products are then added to a “DefaultTableModel” object for display.
10. “deleteProduct”: This method deletes a product from the “products” table based on the provided product ID.
11. “deleteCategory”: This method deletes a category from the “categories” table based on the provided category ID.
12. “getAllCategories”: This method retrieves all categories from the “categories” table and populates them into a “DefaultTableModel” object for displaying in a table.
13. “updateComboBox”: This method retrieves the ID and name of all categories from the “categories” table and populates them into a “JComboBox” for selection.
14. “sellMultipleProducts”: This method is used to sell multiple products at once. It takes two lists as parameters: “productIds” (containing the IDs of the products to sell) and “quantities” (containing the corresponding quantities to sell). It iterates over the lists and updates the quantities of the products in the “products” table using SQL “UPDATE” queries.
Step 2:Creating the AddProductWindow class
Here is the complete code:
package org.ProjectGurukul; import javax.swing.JFrame; import java.awt.GridLayout; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JTextField; import javax.swing.JButton; import java.awt.event.ActionListener; import java.awt.event.ActionEvent; @SuppressWarnings("serial") public class AddProductWindow extends JFrame{ DatabaseConnection connection; private JTextField nameField; private JTextField quantityField; private JTextField priceField; public AddProductWindow(DatabaseConnection connection) { setTitle("ADD Product"); setBounds(100, 100, 440, 300); setDefaultCloseOperation(DISPOSE_ON_CLOSE); this.connection = connection; getContentPane().setLayout(null); JLabel lblName = new JLabel("Name:"); lblName.setBounds(0, 1, 220, 67); getContentPane().add(lblName); nameField = new JTextField(); nameField.setBounds(220, 1, 220, 67); nameField.setColumns(10); getContentPane().add(nameField); JButton btnAdd = new JButton("Add"); btnAdd.setBounds(118, 203, 220, 67); btnAdd.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { try { connection.insertProduct(nameField.getText(),Integer.valueOf(quantityField.getText()),Double.valueOf(priceField.getText())); } catch (NumberFormatException e1) { JOptionPane.showMessageDialog(btnAdd, "Please Enter the numbers only"); e1.printStackTrace(); } } }); JLabel lblQuantity = new JLabel("Quantity"); lblQuantity.setBounds(0, 68, 220, 67); getContentPane().add(lblQuantity); quantityField = new JTextField(); quantityField.setBounds(220, 68, 220, 67); quantityField.setColumns(10); getContentPane().add(quantityField); JLabel lblPrice = new JLabel("Price:"); lblPrice.setBounds(0, 135, 220, 67); getContentPane().add(lblPrice); priceField = new JTextField(); priceField.setBounds(220, 135, 220, 67); priceField.setColumns(10); getContentPane().add(priceField); getContentPane().add(btnAdd); } }
Step 3: Creating the UI for our application in class “InventoryManagement.java”:
You can use WindowBuilder extension in Eclipse
Here’s the complete code for InventoryManagement.java:
package org.ProjectGurukul; import java.awt.EventQueue; import javax.swing.JFrame; import javax.swing.JOptionPane; import javax.swing.JTabbedPane; import java.awt.BorderLayout; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JComboBox; import javax.swing.table.DefaultTableModel; import java.awt.GridLayout; import java.awt.Color; import javax.swing.JButton; import java.awt.event.ActionListener; import java.util.ArrayList; import java.util.Arrays; import java.awt.event.ActionEvent; import javax.swing.JToggleButton; import javax.swing.JLabel; import javax.swing.JTextField; import javax.swing.BoxLayout; public class InventoryManagement { DatabaseConnection connection = new DatabaseConnection("InventoryDB"); private JFrame frame; private JTable purchaseTable; private DefaultTableModel producTableModel = new DefaultTableModel(new Object[] {"ID","Name","Quantity","Price","Created","Updated"}, 0); private DefaultTableModel categoriesTableModel = new DefaultTableModel(new Object[] {"ID","Name"}, 0); private DefaultTableModel inventotyTableModel = new DefaultTableModel( new Object[] { "ID", "Name", "Quantity","price", "Created", "Updated" },0 ); private JTextField idField; private JTextField quantityField; private JTable invntoryTable; private JTextField productIDField; private JTextField categIDField; private JTextField pidField; private JTextField quantitiesField; /** * Launch the application. */ public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { try { InventoryManagement window = new InventoryManagement(); window.frame.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } }); } /** * Create the application. */ public InventoryManagement() { connection.createSchema(); initialize(); } /** * Initialize the contents of the frame. */ private void initialize() { frame = new JFrame(); frame.setBounds(100, 100, 700, 550); frame.setTitle("Inventory Management by ProjectGurukul"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.getContentPane().setLayout(null); JTabbedPane tabbedPane = new JTabbedPane(JTabbedPane.TOP); tabbedPane.setBounds(0, 0, 700, 520); frame.getContentPane().add(tabbedPane); JPanel purchasePanel = new JPanel(); tabbedPane.addTab("Purchase", null, purchasePanel, null); purchasePanel.setLayout(null); JPanel buttonsPanel = new JPanel(); buttonsPanel.setBackground(new Color(143, 240, 164)); buttonsPanel.setBounds(0, 425, 700, 70); purchasePanel.add(buttonsPanel); buttonsPanel.setLayout(new GridLayout(1, 0, 5, 5)); JButton btnDeleteCategory = new JButton("Delete Category"); btnDeleteCategory.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String id = (String)JOptionPane.showInputDialog(btnDeleteCategory, "Enter the category Id to Delete:"); try { connection.deleteCategory(Integer.valueOf(id)); } catch (NumberFormatException e1) { JOptionPane.showMessageDialog(btnDeleteCategory, "Invalid ID"); e1.printStackTrace(); } } }); buttonsPanel.add(btnDeleteCategory); JButton btnDeleteProduct = new JButton("Delete Product"); btnDeleteProduct.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String id = (String)JOptionPane.showInputDialog(btnDeleteProduct, "Enter the Product Id to Delete:"); try { connection.deleteProduct(Integer.valueOf(id)); } catch (NumberFormatException e1) { JOptionPane.showMessageDialog(btnDeleteCategory, "Invalid ID"); e1.printStackTrace(); } } }); buttonsPanel.add(btnDeleteProduct); JButton btnAddNewCategory = new JButton("Add New Category"); btnAddNewCategory.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String name = (String)JOptionPane.showInputDialog(btnAddNewCategory, "Enter the category name to add:"); connection.insertCategory(name); } }); buttonsPanel.add(btnAddNewCategory); JButton btnAddNewProduct = new JButton("Add New Product"); btnAddNewProduct.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { AddProductWindow window = new AddProductWindow(connection); window.setVisible(true); } }); buttonsPanel.add(btnAddNewProduct); JToggleButton tglbtn = new JToggleButton("Products"); tglbtn.setBounds(0, 0, 167, 25); tglbtn.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(tglbtn.getText() == "Products") { tglbtn.setText("Categories"); purchaseTable.setModel(categoriesTableModel); connection.getAllCategories(categoriesTableModel); }else { tglbtn.setText("Products"); purchaseTable.setModel(producTableModel); connection.getAllProducts(producTableModel); } } }); purchasePanel.add(tglbtn); JScrollPane scrollPane = new JScrollPane(); scrollPane.setBounds(0, 25, 700, 300); purchasePanel.add(scrollPane); purchaseTable = new JTable(producTableModel); scrollPane.setViewportView(purchaseTable); JLabel lblId = new JLabel("ID:"); lblId.setBounds(10, 393, 70, 25); purchasePanel.add(lblId); idField = new JTextField(); idField.setBounds(78, 393, 114, 25); purchasePanel.add(idField); idField.setColumns(10); JLabel lblQuantity = new JLabel("Quantity:"); lblQuantity.setBounds(210, 393, 70, 25); purchasePanel.add(lblQuantity); quantityField = new JTextField(); quantityField.setColumns(10); quantityField.setBounds(298, 393, 114, 25); purchasePanel.add(quantityField); JButton btnPurchase = new JButton("Purchase"); btnPurchase.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { try { connection.purchaseProduct(Integer.valueOf(idField.getText()), Integer.valueOf(quantityField.getText())); } catch (NumberFormatException e1) { JOptionPane.showMessageDialog(btnPurchase, "Please enter Valid values"); e1.printStackTrace(); } } }); btnPurchase.setBounds(438, 393, 117, 25); purchasePanel.add(btnPurchase); JLabel lblProduc = new JLabel("Product Id:"); lblProduc.setBounds(12, 337, 100, 25); purchasePanel.add(lblProduc); productIDField = new JTextField(); productIDField.setColumns(10); productIDField.setBounds(107, 337, 114, 25); purchasePanel.add(productIDField); JLabel lblCategoryId = new JLabel("Category Id:"); lblCategoryId.setBounds(222, 337, 100, 25); purchasePanel.add(lblCategoryId); categIDField = new JTextField(); categIDField.setColumns(10); categIDField.setBounds(323, 337, 114, 25); purchasePanel.add(categIDField); JButton btnSet = new JButton("Set"); btnSet.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { try { connection.assignCategoryToProduct(Integer.valueOf(productIDField.getText()),Integer.valueOf(categIDField.getText())); } catch (NumberFormatException e1) { JOptionPane.showMessageDialog(btnSet, "Invalid ID"); e1.printStackTrace(); } } }); btnSet.setBounds(453, 337, 70, 25); purchasePanel.add(btnSet); JPanel inventoryPanel = new JPanel(); tabbedPane.addTab("Inventory & Sales", null, inventoryPanel, null); inventoryPanel.setLayout(null); JScrollPane scrollPane_1 = new JScrollPane(); scrollPane_1.setLocation(0, 25); scrollPane_1.setSize(700, 350); inventoryPanel.add(scrollPane_1); invntoryTable = new JTable(inventotyTableModel); scrollPane_1.setViewportView(invntoryTable); JLabel lblSelectCategory = new JLabel("Select Category"); lblSelectCategory.setBounds(0, 0, 125, 15); inventoryPanel.add(lblSelectCategory); JComboBox<String> categoryComboBox = new JComboBox<String>(); categoryComboBox.setBounds(125, 0, 125, 24); inventoryPanel.add(categoryComboBox); connection.updateComboBox(categoryComboBox); JLabel lblEnterPidSeparated = new JLabel("Enter PIDs separated by \",\""); lblEnterPidSeparated.setBounds(10, 387, 200, 15); inventoryPanel.add(lblEnterPidSeparated); pidField = new JTextField(); pidField.setBounds(263, 387, 420, 25); inventoryPanel.add(pidField); pidField.setColumns(10); JLabel lblEnterQuantitiesSeparated = new JLabel("Enter Quantities separated by \",\""); lblEnterQuantitiesSeparated.setBounds(0, 414, 240, 15); inventoryPanel.add(lblEnterQuantitiesSeparated); quantitiesField = new JTextField(); quantitiesField.setColumns(10); quantitiesField.setBounds(263, 414, 420, 25); inventoryPanel.add(quantitiesField); JButton btnSellProducts = new JButton("Sell Products"); btnSellProducts.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { ArrayList<String> pidArrayList = new ArrayList<>(Arrays.asList(pidField.getText().split(","))); ArrayList<String> quantArrayList = new ArrayList<>(Arrays.asList(quantitiesField.getText().split(","))); JOptionPane.showMessageDialog(btnSellProducts, connection.sellMultipleProducts(pidArrayList, quantArrayList)); } }); btnSellProducts.setBounds(254, 451, 130, 25); inventoryPanel.add(btnSellProducts); categoryComboBox.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String id = (String)categoryComboBox.getSelectedItem(); id = id.substring(0, id.lastIndexOf("|")); connection.getProductsByCategory(Integer.valueOf(id),inventotyTableModel); } }); } }
Java Inventory Management System Output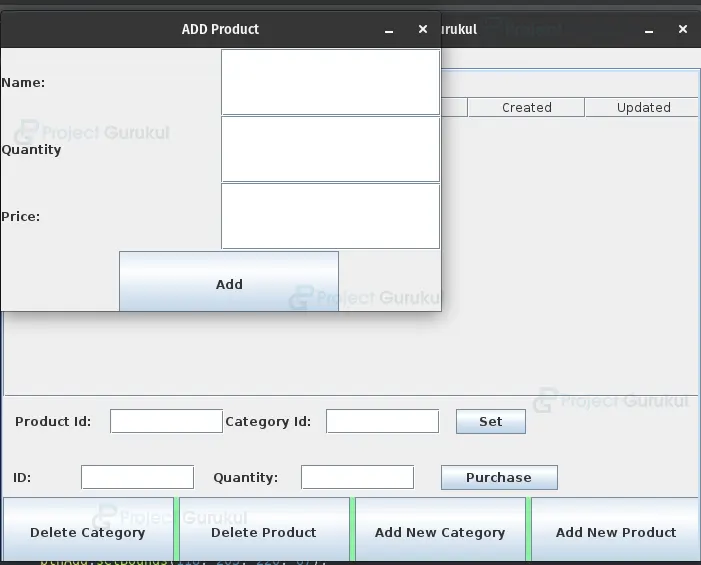
Summary
This project provides a functional Inventory Management system GUI implemented in Java Swing. It allows users to perform various tasks such as adding new products and categories, assigning categories to products, purchasing products, and tracking inventory and sales. The code utilizes a database connection for storing and retrieving data related to products, categories, and transactions. By studying this code, you can learn how to create a GUI-based inventory management application in Java and integrate it with a database for data persistence.
If you are Happy with ProjectGurukul, do not forget to make us happy with your positive feedback on Google | Facebook
How to run this on eclipse please guide . Urgent help required. Thank you in advance.
Can you include user authentication for data privacy? And also can it be more analytical so that the previous data and current data can be used for future predictions